Comparing ast-grep and jscodeshift
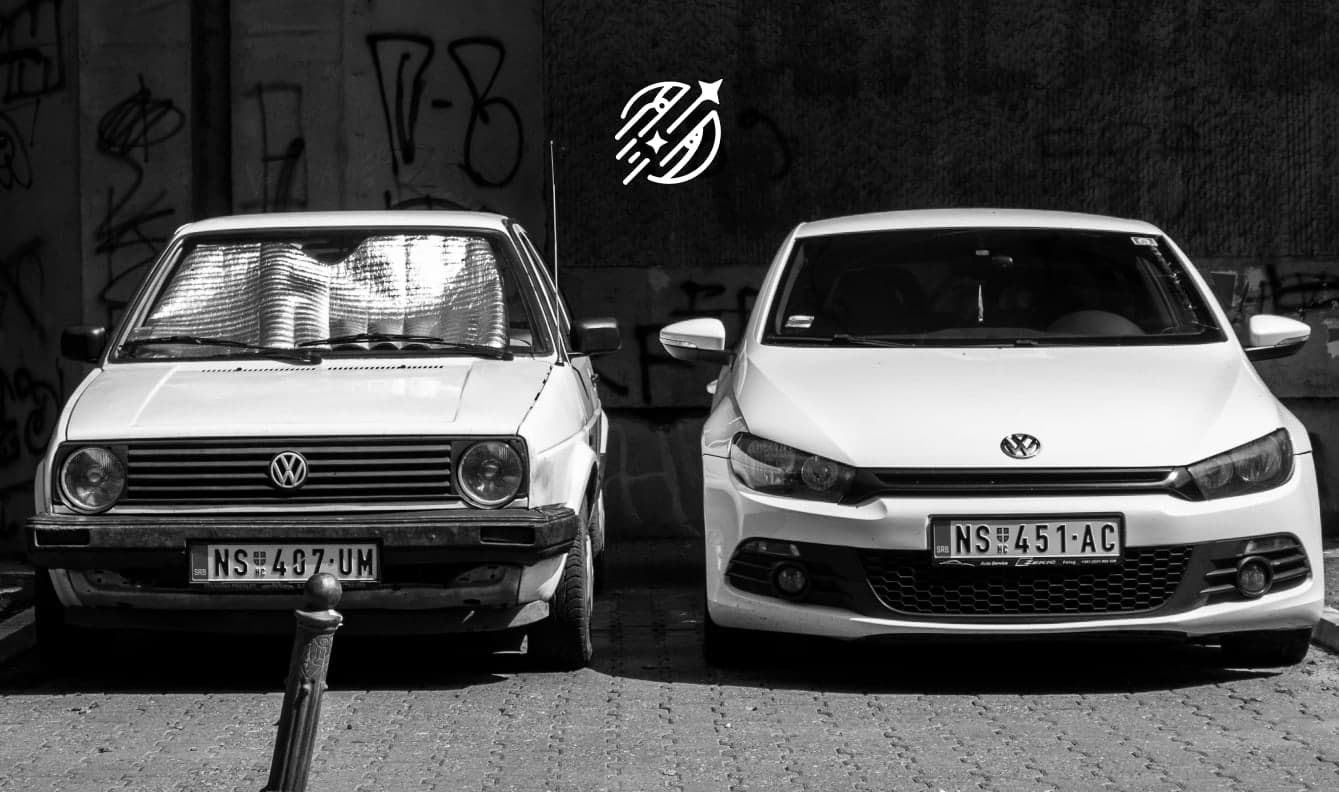
When it comes to programmatically modifying JavaScript and TypeScript code, two popular tools stand out: ast-grep and jscodeshift. Both allow developers to work with the abstract syntax tree (AST) of their code to apply transformations efficiently, but they take different approaches and serve different use cases. In this post, we'll compare these two tools in terms of functionality, performance, ease of use, and best use cases.
What is ast-grep?
ast-grep is a powerful pattern-matching and code transformation tool inspired by grep
, but built for ASTs. It provides a declarative approach to finding and replacing patterns in source code, making it a great choice for linting, refactoring, and enforcing coding standards.
Key Features of ast-grep:
- Pattern-based matching using YAML or JSON
- Multi-language support (JS, TS, Rust, Python, etc.)
- Fast execution powered by Rust
- Declarative syntax makes it easy to apply transformations without writing complex traversal logic
- CLI-based and integrates well with CI/CD workflows
- Flexible query language with regex-style pattern matching
Example Usage:
rule: pattern: 'console.log($ARG)' fix: 'console.debug($ARG)'
This rule finds all console.log
statements and replaces them with console.debug
.
What is jscodeshift?
jscodeshift is a tool from Facebook designed specifically for JavaScript and TypeScript transformations. It provides an API based on recast and babel, allowing developers to traverse and modify ASTs using JavaScript code.
Key Features of jscodeshift:
- Imperative transformation API using JavaScript
- Built on recast, preserving original code formatting
- Works well for large-scale refactoring
- Supports custom codemods for targeted migrations
- Integrates with Babel plugins for advanced transformations
- CLI-based and scriptable for automation
Example Usage:
export default function transformer(fileInfo, api) { const j = api.jscodeshift; return j(fileInfo.source) .find(j.CallExpression, { callee: { object: { name: 'console' }, property: { name: 'log' } } }) .replaceWith((path) => j.callExpression( j.memberExpression(j.identifier('console'), j.identifier('debug')), path.value.arguments ) ) .toSource(); }
This script finds console.log
calls and replaces them with console.debug
.
Comparing ast-grep vs jscodeshift
1. Ease of Use
- ast-grep: Easier to use for simple transformations and linting due to its pattern-matching approach. Users can define rules declaratively in YAML.
- jscodeshift: Requires writing JavaScript code and understanding AST traversal, which has a steeper learning curve.
2. Performance
- ast-grep: Extremely fast since it’s written in Rust, making it ideal for large codebases.
- jscodeshift: Slower in comparison because it’s JavaScript-based and relies on Babel/Recast.
3. Customization and Flexibility
- ast-grep: Works best for structured, declarative searches but may be less flexible for complex transformations.
- jscodeshift: More powerful for deep transformations, allowing full control over AST manipulation.
4. Code Formatting and Preservation
- ast-grep: May not always preserve original formatting when applying fixes.
- jscodeshift: Uses recast, which maintains original code style and comments.
5. Use Cases
Use Case | ast-grep | jscodeshift |
---|---|---|
Finding code patterns | ✅ | ✅ |
Enforcing linting rules | ✅ | ❌ |
Large-scale refactoring | ✅ | ✅ |
Custom codemods | ❌ | ✅ |
Performance-sensitive applications | ✅ | ❌ |
CI/CD integration | ✅ | ✅ |
When to Use Which?
- Use ast-grep if you need a fast, declarative tool to enforce code patterns, linting rules, or perform simple code transformations across multiple languages.
- Use jscodeshift if you need fine-grained control over JavaScript and TypeScript transformations, especially for large-scale migrations and codemods.
Conclusion
Both ast-grep and jscodeshift are powerful tools for working with JavaScript and TypeScript ASTs, but they cater to different needs. If you’re looking for a fast, pattern-based approach, ast-grep is your best bet. If you need to perform complex refactoring and maintain formatting, jscodeshift is the way to go. Choosing the right tool depends on your use case and how much control you need over your code transformations.
Which tool do you prefer for your code modifications? Let us know in the comments!